
星期四, 12月 28, 2006
星期一, 12月 25, 2006
星期一, 12月 18, 2006
sample code of a class with static methods
public class RoundStuff
{
public static final double PI=3.14159;
public static double area(double radius)
{
return (PI*radius*radius);
}
public static double volume(double radius)
{
return((4.0/3.0)*PI*radius*radius*radius);
}
}
{
public static final double PI=3.14159;
public static double area(double radius)
{
return (PI*radius*radius);
}
public static double volume(double radius)
{
return((4.0/3.0)*PI*radius*radius*radius);
}
}
Lab Static Method, Part II
(1st ed.) Do Project 3 of Chap 5. Define a Complex class and write a program to compute (2+3i)+(4+5i) in Java.
(2nd ed.) Do Project 7 of Chap 5. Define a Complex class and write a program to compute (2+3i)+(4+5i) in Java.
Note:
Implement a static method and a nonstatic method using overloading. The results of these two methods should be the same.
(2nd ed.) Do Project 7 of Chap 5. Define a Complex class and write a program to compute (2+3i)+(4+5i) in Java.
Note:
Implement a static method and a nonstatic method using overloading. The results of these two methods should be the same.
Lab Static Method
Study Display 5.2.
Using static variables and static methods to implement the class Fibonacci such that
the first call to Fibonacci.next()
returns 2, the second returns 3, and then 5, and so on.
Using static variables and static methods to implement the class Fibonacci such that
the first call to Fibonacci.next()
returns 2, the second returns 3, and then 5, and so on.
星期二, 12月 12, 2006
星期一, 12月 11, 2006
隨堂上機考 (1) Pass
9226122,
9226133
9226143
9226144
9226251
9322326
9326101
9326107
9326119
9326151
9326205
9326243
9326252
9326253
9326254
9326258
9326264
9326319
9326324
9326330
9326344
9326346
9326349
9326359
9326363
9326366
9426344
9226133
9226143
9226144
9226251
9322326
9326101
9326107
9326119
9326151
9326205
9326243
9326252
9326253
9326254
9326258
9326264
9326319
9326324
9326330
9326344
9326346
9326349
9326359
9326363
9326366
9426344
隨堂上機考 (2)
Write a program to implement a method that can do additions of 2 fractions. You will implement a class called Fraction consisting of a numerator and a denominator. The additions of
2 fractions should be equal to a fraction.
Use 1/2+1/3 as the test.
Hints:
Fraction f1, f2;
f1.add(f2);
2 fractions should be equal to a fraction.
Use 1/2+1/3 as the test.
Hints:
Fraction f1, f2;
f1.add(f2);
隨堂上機考 (1)
Define a class called Counter whose objects count things. An object of this class records a count that is a nonnegative integer. Include methods to set the counter to 0, to increase the count by 1, and to decrease the count by 1. Include an accessor method that returns the current count value and a method that outputs the count to the screen. Write a program to test |
星期五, 12月 08, 2006
星期一, 12月 04, 2006
大三專題說明
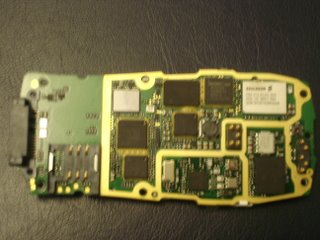
張耀仁老師
專題題目
- PDA 無線定位軟體實作與應用 (Project Navizon)
- 行動義工軟體開發 (Programming on PDA)
- 網站程式設計
1/3/2007
1/19/2007
老師會談時間
- 建議先 email 與我約時間 (yjchang at cycu.edu.tw)
Lab Temperature Project
Do Temperature Project, which is Project 7 (2nd ed.) or Project 3 (1st ed.).
Lab Java Constructor
Use Display 4.14 to call 4.13 (2nd ed.) or
Display 4.12 to call 4.11 (1st ed.).
After you finish the above, try the following
Date birthday = new Date("Jan",1,2000);
birthday.Date("Feb",1,2000);
birthday.setDate("Feb",1,2000);
birthday=new Date("Mar",1,2000);
Display 4.12 to call 4.11 (1st ed.).
After you finish the above, try the following
Date birthday = new Date("Jan",1,2000);
birthday.Date("Feb",1,2000);
birthday.setDate("Feb",1,2000);
birthday=new Date("Mar",1,2000);
星期一, 11月 27, 2006
Java Overloading
Study Display 4.11 and use Display 4.12 to call 4.11. (2nd ed.)
Study Display 4.9 and use Display 4.10 to call 4.9 (1st ed.)
Study Display 4.9 and use Display 4.10 to call 4.9 (1st ed.)
Lab Class Definitions III
Do Display 4.7 (2nd ed.) or 4.5 (1st ed.). Then use Display 4.8 to call 4.7.
Requirements
1. The method setDate has the parameter as setDate(int month, int day, int year).
What kind of changes should be made in its body of codes?
Requirements
1. The method setDate has the parameter as setDate(int month, int day, int year).
What kind of changes should be made in its body of codes?
星期一, 11月 20, 2006
Lab Class Definitions II
Study Display 4.2 & Display 4.4 (2nd ed.) or Display 4.2 & Display 4.3 (1st ed.) and then
1. comment out date.setDate(6, 17, year); by // date.setDate(6, 17, year);
2. At the next line below, add date.readInput();
3. Run the program again. Fix any problems you may encouter along the way.
4. At the last line of your program, add System.out.println(date.month);
and see what happens. Why?
1. comment out date.setDate(6, 17, year); by // date.setDate(6, 17, year);
2. At the next line below, add date.readInput();
3. Run the program again. Fix any problems you may encouter along the way.
4. At the last line of your program, add System.out.println(date.month);
and see what happens. Why?
星期一, 11月 06, 2006
Java 2
Java 2 分成 Java 2 Platform Enterprise Edition(簡稱 J2EE)、Java 2 Platform Standard Edition(簡稱 J2SE)、Java 2 Platform Micro Edition(簡稱 J2ME)。J2EE 適用於伺服器,目前已經成為企業運算、 電子商務等領域中相當熱門的技術;J2SE 適用於一般的電腦;J2ME 適用於消費性電子產品。
星期二, 10月 31, 2006
遲交不計分清單
<已經更改過名單>
處理原則
Lab Get Familiar with Java StringLab 10-2 (1) Simple Java Expression Lab 9-25 (1) Get familiar with JBuilder
Lab: Using Firefox
Homework 1
Lab Blogging
處理原則
- Lab 超過下課24小時未交, 不予記分. 未交 Lab, 不僅該 Lab 不記分, 且扣平時成績.
- Homework 超過繳交時間(上課前) , 不予記分
- 如有任何問題請在以下Comment 發表, 助教認同原因後方可註銷.
- Lab 與 Homework 的重要性參看課程大綱
Lab Get Familiar with Java StringLab 10-2 (1) Simple Java Expression Lab 9-25 (1) Get familiar with JBuilder
Lab: Using Firefox
Homework 1
Lab Blogging
星期一, 10月 30, 2006
Lab Exponential Funtion
Do Project 7 of Chap. 3. (2nd ed.) or Project 4 (1st ed.)
Hint: You don't have to use nested loops.
Hint: You don't have to use nested loops.
Lab Fibonacci numbers
Do Project 3.3 Fibonacci numbers.
List the first 100 numbers and the ratio of
a number to its previous number.
Want to know more about Fibonacci number
List the first 100 numbers and the ratio of
a number to its previous number.
Want to know more about Fibonacci number
星期一, 10月 23, 2006
Homework 10-23-2006 Finding the max and the min
Based on your study of Display 3.8, write a code to find the max and min of a list of number.
For example, given 1,3,5, and9, the max is 9 and the min is 1.
Your program should be able to process a list of any length.
For example, given 1,3,5, and9, the max is 9 and the min is 1.
Your program should be able to process a list of any length.
Lab Tax Calculation
Study Display 3.1. Based on the income tax rate in Taiwan,
calculate the income tax of a person whose annual income is 1,000,000.
calculate the income tax of a person whose annual income is 1,000,000.
星期日, 10月 22, 2006
老師時間
張老師開放以下時間提供同學當面發問, 地點電子大樓 512 老師研究室
星期一 下午 2:00~4:00
星期五 上午 11:00~12:00, 下午 4:10-5:00
星期六 上午 10:00~12:00
星期一 下午 2:00~4:00
星期五 上午 11:00~12:00, 下午 4:10-5:00
星期六 上午 10:00~12:00
9/18~10/22 遲交不記分清單
處理原則
Lab Get Familiar with Java StringLab 10-2 (1) Simple Java Expression Homework 9-25-2006Lab 9-25 (1) Get familiar with JBuilderLab: Using FirefoxHomework 1Lab Blogging
- Lab 超過下課24小時未交, 不予記分. 未交 Lab, 不僅該 Lab 不記分, 且扣平時成績.
- Homework 超過繳交時間(上課前) , 不予記分
- 如有任何問題請在以下Comment 發表, 助教認同原因後方可註銷.
- Lab 與 Homework 的重要性參看課程大綱
Lab Get Familiar with Java StringLab 10-2 (1) Simple Java Expression Homework 9-25-2006Lab 9-25 (1) Get familiar with JBuilderLab: Using FirefoxHomework 1Lab Blogging
星期一, 10月 16, 2006
Lab Scanner
Do Display 2.6 on Page 79.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
Change
Scanner keyboard= new Scanner(System.in);
into
BufferedReader keyboard= new BufferedReader(new InputStreamReader(System.in));
String inputString = keyboard.readLine();
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
Change
Scanner keyboard= new Scanner(System.in);
into
BufferedReader keyboard= new BufferedReader(new InputStreamReader(System.in));
String inputString = keyboard.readLine();
Lab window input dialog
Use the following command to generate a window input dialog
String myString=JOptionPane.showInputDialog("Enter a number: ");
use the following command to convert a string to a number
int myNumber = Integer.parseInt(myString);
and finally use the following command to print the input number to the console
System.out.println("The number is "+ myNumber);
Don't forget the following import command
import javax.swing.JOptionPane;
so that Java can recognize JOptionPane library.
String myString=JOptionPane.showInputDialog("Enter a number: ");
use the following command to convert a string to a number
int myNumber = Integer.parseInt(myString);
and finally use the following command to print the input number to the console
System.out.println("The number is "+ myNumber);
Don't forget the following import command
import javax.swing.JOptionPane;
so that Java can recognize JOptionPane library.
星期日, 10月 15, 2006
Lab Get Familiar with Java String
Display 1.7 of Chap. 1
Project 5 of Chap. 1
"tell me and I'll forget; show me and I may remember; involve me and I'll understand"
Project 5 of Chap. 1
"tell me and I'll forget; show me and I may remember; involve me and I'll understand"
星期五, 10月 06, 2006
星期一, 10月 02, 2006
星期六, 9月 30, 2006
有修Java的同學請注意一下
1.文章的出處請記得標明
2.請別直接複製別人的網頁 , 因為複製過多別人的網頁,
會被評為垃圾部落格 , 到時候就無法發表文章
3.電子四甲的鍾兆豐同學 , 你的部落格好像遺失 , 無法看到 , 請跟我回應一下 , 謝謝
2.請別直接複製別人的網頁 , 因為複製過多別人的網頁,
會被評為垃圾部落格 , 到時候就無法發表文章
3.電子四甲的鍾兆豐同學 , 你的部落格好像遺失 , 無法看到 , 請跟我回應一下 , 謝謝
星期一, 9月 25, 2006
Lab 10-2 (1) Simple Java Expression
Do Project 4 on Page 56. (2nd Edition)
Do Project 1 on Page 54. (1st Edition)
Do Project 1 on Page 54. (1st Edition)
Homework 9-25-2006
"tell me and I'll forget; show me and I may remember; involve me and I'll understand"
Homework Problems due 10/2 18:50
1. Explain bytecode, JVM
2. Explain class, object
3. Reading Assignments:
Read 1.1, 1.2, 1.3 of Textbook
4.1 Write a Java program as follows:
Let i=2;
Print i;
Print 2 * (i++);
Print i;
Ans: 2, 4, 3
4.2 Write a Java program as follows:
Let i=2;
Print i;
Print 2 * (++i);
Print i;
Ans: 2, 6, 3
4.3 Write a Java program as follows:
Let m=7, n=2;
Print (double) m/n;
Print m/ (double)n;
Ans: 3.5, 3.5
Homework Problems due 10/2 18:50
1. Explain bytecode, JVM
2. Explain class, object
3. Reading Assignments:
Read 1.1, 1.2, 1.3 of Textbook
4.1 Write a Java program as follows:
Let i=2;
Print i;
Print 2 * (i++);
Print i;
Ans: 2, 4, 3
4.2 Write a Java program as follows:
Let i=2;
Print i;
Print 2 * (++i);
Print i;
Ans: 2, 6, 3
4.3 Write a Java program as follows:
Let m=7, n=2;
Print (double) m/n;
Print m/ (double)n;
Ans: 3.5, 3.5
星期一, 9月 18, 2006
Homework 1
1. List at least 5 features of the Java Language.
2. List at least 5 applications of Java. You must provide the references you used. We recommend Google Search engine.
2. List at least 5 applications of Java. You must provide the references you used. We recommend Google Search engine.
Lab: Using Firefox
Outlines of Lab
1. download Firefox 1.5
2. control font size
3. tabbed browsing
4. subscribe to the course blog
5. Using bookmarks to test RSS feed
Help
Hands on
1. Easy control of font size (try ctrl+ & ctrl- as many times as you like)
2. tabbed browsing (try ctrlT)
3. RSS feed, and support of Blog. Use the RSS feed from http://iapblog.blogspot.com/
to subscribe to it. Also subscribe to your own blog.
Note:
If your Firefox didn't work, you should check settings about proxy. It should be set as proxy.cycu.edu.tw at port 3128.
1. download Firefox 1.5
2. control font size
3. tabbed browsing
4. subscribe to the course blog
5. Using bookmarks to test RSS feed
Help
Hands on
1. Easy control of font size (try ctrl+ & ctrl- as many times as you like)
2. tabbed browsing (try ctrlT)
3. RSS feed, and support of Blog. Use the RSS feed from http://iapblog.blogspot.com/
to subscribe to it. Also subscribe to your own blog.
Note:
If your Firefox didn't work, you should check settings about proxy. It should be set as proxy.cycu.edu.tw at port 3128.
星期四, 9月 14, 2006
Lab: Blogging
1. 至 http://www.blogger.com 申請, 請以你的學號 (s開頭) 申請免費帳號。主旨欄請用以下格式 "Java作業一" "Java Lab 1"
以加速助教登錄作業成績。
2. 請將你的作業寫在你的 blog, 然後到
Homework 或 Lab 的Comment 登錄作業blog網址就可以了.
請勿將作業直接寫在 comment
以加速助教登錄作業成績。
2. 請將你的作業寫在你的 blog, 然後到
Homework 或 Lab 的Comment 登錄作業blog網址就可以了.
請勿將作業直接寫在 comment
星期五, 6月 23, 2006
星期一, 6月 12, 2006
Lab Hanoi Tower
The pseudocode for Hanoi Tower is as follows:
Write the Java program based on the pseudocode in the above.
Solve(N, Src, Aux, Dst)
if N is 0 return
Solve(N-1, Src, Dst, Aux)
Move N from Src to Dst
Solve(N-1, Aux, Src, Dst)
Write the Java program based on the pseudocode in the above.
Lab Recursive Method
Write a recursive method that computes the sum of squares from 1 to n, given an interger n.
星期一, 5月 29, 2006
Homework 5-29-2006 Lab Squared Numbers in an Array
Write a program that given an array of integers, return the squares of the array.
The sqaures are computed in a separate method rather than in the main method.
The sqaures are computed in a separate method rather than in the main method.
Cookie
Lab modular sorting
Write a sort method which takes a double array as parameter
and returns the sorted array. Call this method in a main program.
Hint: The lab is a rewriting of Lab Sorting
to make the sorting procedure a reusable method.
and returns the sorted array. Call this method in a main program.
Hint: The lab is a rewriting of Lab Sorting
to make the sorting procedure a reusable method.
Cookie
星期一, 5月 15, 2006
Lab Sorting
Study Display 6.1, and then write a program that can sort 5 numbers in ascending order. The 5 numbers are input by the user.
星期一, 5月 08, 2006
Lab Static Method, Part II
(1st ed.) Do Project 3 of Chap 5. Define a Complex class and write a program to compute (2+3i)+(4+5i) in Java. |
星期一, 5月 01, 2006
Lab Static Method
Study Display 5.2.
Using static variables and static methods to implement the class Fibonacci such that
the first call to Fibonacci.next()
returns 2, the second returns 3, and then 5, and so on.
Using static variables and static methods to implement the class Fibonacci such that
the first call to Fibonacci.next()
returns 2, the second returns 3, and then 5, and so on.
Lab Java Constructor
Use Display 4.14 to call 4.13 (2nd ed.) or
Display 4.12 to call 4.11 (1st ed.).
After you finish the above, try the following
Date birthday = new Date("Jan",1,2000);
birthday.Date("Feb",1,2000);
birthday.setDate("Feb",1,2000);
birthday=new Date("Mar",1,2000);
Display 4.12 to call 4.11 (1st ed.).
After you finish the above, try the following
Date birthday = new Date("Jan",1,2000);
birthday.Date("Feb",1,2000);
birthday.setDate("Feb",1,2000);
birthday=new Date("Mar",1,2000);
星期二, 4月 25, 2006
Homework 04-25-2006 (Java Overloading)
Study Display 4.11 and use Display 4.12 to call 4.11. (2nd ed.)
Study Display 4.9 and use Display 4.10 to call 4.9 (1st ed.)
Study Display 4.9 and use Display 4.10 to call 4.9 (1st ed.)
星期一, 4月 10, 2006
Lab Class Definitions III
Do Display 4.7 (2nd ed.) or 4.5 (1st ed.). Then use Display 4.8 to call 4.7.
Requirements
1. The method setDate has the parameter as setDate(int month, int day, int year).
What kind of changes should be made in its body of codes?
Requirements
1. The method setDate has the parameter as setDate(int month, int day, int year).
What kind of changes should be made in its body of codes?
Cookie
星期一, 3月 27, 2006
Lab Class Definitions II
Study Display 4.2 & Display 4.4 (2nd ed.) or Display 4.2 & Display 4.3 (1st ed.) and then
1. comment out date.setDate(6, 17, year); by // date.setDate(6, 17, year);
2. At the next line below, add date.readInput();
3. Run the program again. Fix any problems you may encouter along the way.
4. At the last line of your program, add System.out.println(date.month);
and see what happens. Why?
1. comment out date.setDate(6, 17, year); by // date.setDate(6, 17, year);
2. At the next line below, add date.readInput();
3. Run the program again. Fix any problems you may encouter along the way.
4. At the last line of your program, add System.out.println(date.month);
and see what happens. Why?
星期一, 3月 20, 2006
Lab Finding max from a list of numbers
Write a program that reads 10 numbers from the console.
Pick the largest bumber from the list.
Pick the largest bumber from the list.
Lab Exponential Funtion
Do Project 7 of Chap. 3. (2nd ed.) or Project 4 (1st ed.)
Hint: This lab gets you familiarized with nested for loops.
Hint: This lab gets you familiarized with nested for loops.
Homework 3-20-2006 Lab Fibonacci Numbers
Do Project 3.3 Fibonacci numbers.
List the first 100 numbers and the ratio of
a number to its previous number.
Want to know more about Fibonacci number
List the first 100 numbers and the ratio of
a number to its previous number.
Want to know more about Fibonacci number
星期一, 3月 13, 2006
星期一, 3月 06, 2006
Lab Finding the max and the min
Based on your study of Display 3.8, write a code to find the max and min of a list of number.
Lab Tax Calculation
Study Display 3.1. Based on the income tax rate in Taiwan,
calculate the income tax of a person whose annual income is 1,000,000.
calculate the income tax of a person whose annual income is 1,000,000.
Lab Scanner
Do Display 2.6 on Page 79.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
Change
Scanner keyboard= new Scanner(System.in);
into
BufferedReader keyboard= new BufferedReader(new InputStreamReader(System.in));
String inputString = keyboard.readLine();
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
Change
Scanner keyboard= new Scanner(System.in);
into
BufferedReader keyboard= new BufferedReader(new InputStreamReader(System.in));
String inputString = keyboard.readLine();
星期一, 2月 27, 2006
Homework 03/06/2006 Lab JOptionPane (Chapter 2)
Use the following command to generate a window input dialog
String myString=JOptionPane.showInputDialog("Enter a number: ");
use the following command to convert a string to a number
int myNumber = Integer.parseInt(myString);
and finally use the following command to print the input number to the console
System.out.println("The number is "+ myNumber);
Don't forget the following import command
import javax.swing.JOptionPane;
so that Java can recognize JOptionPane library.
String myString=JOptionPane.showInputDialog("Enter a number: ");
use the following command to convert a string to a number
int myNumber = Integer.parseInt(myString);
and finally use the following command to print the input number to the console
System.out.println("The number is "+ myNumber);
Don't forget the following import command
import javax.swing.JOptionPane;
so that Java can recognize JOptionPane library.
Lab Get Familiar with Java String
Display 1.7 of Chap. 1
Project 5 of Chap. 1
"tell me and I'll forget; show me and I may remember; involve me and I'll understand"
Project 5 of Chap. 1
"tell me and I'll forget; show me and I may remember; involve me and I'll understand"
Homework 02/27/2006
Reading Assignments
1. Chapter 1
Homework
1. Project 1 on Page 55.
"nothing builds self-esteem and self-confidence like accomplishment"
1. Chapter 1
Homework
1. Project 1 on Page 55.
"nothing builds self-esteem and self-confidence like accomplishment"
星期一, 2月 20, 2006
2-20-2006 Homework
Homework Problems
1. Explain bytecode, JVM
2. Explain class, object
3. Let i=2;
Print i;
Print 2 * (i++);
Print i;
Ans: 2, 4, 3
4. Let i=2;
Print i;
Print 2 * (++i);
Print i;
Ans: 2, 6, 3
5. Let m=7, n=2;
Print (double) m/n;
Print m/ (double)n;
Ans: 3.5, 3.5
Reading Assignments
Read 1.1, 1.2, 1.3
1. Explain bytecode, JVM
2. Explain class, object
3. Let i=2;
Print i;
Print 2 * (i++);
Print i;
Ans: 2, 4, 3
4. Let i=2;
Print i;
Print 2 * (++i);
Print i;
Ans: 2, 6, 3
5. Let m=7, n=2;
Print (double) m/n;
Print m/ (double)n;
Ans: 3.5, 3.5
Reading Assignments
Read 1.1, 1.2, 1.3
星期六, 1月 14, 2006
Lab: Using Firefox
1. download Firefox 1.5
2. control font size
3. tabbed browsing
4. subscribe to the course blog
5. Using bookmarks to test RSS feed
Help
2. control font size
3. tabbed browsing
4. subscribe to the course blog
5. Using bookmarks to test RSS feed
Help
Lab: Blogging
1. 至 http://www.blogger.com 申請, 請以你的學號 (s開頭) 申請免費帳號。主旨欄請用以下格式 "Java作業一" "Java Lab 1"
以加速助教登錄作業成績。
2. 請將你的作業寫在你的 blog, 然後到
Homework 或 Lab 的Comment 登錄作業blog網址就可以了.
請勿將作業直接寫在 comment
以加速助教登錄作業成績。
2. 請將你的作業寫在你的 blog, 然後到
Homework 或 Lab 的Comment 登錄作業blog網址就可以了.
請勿將作業直接寫在 comment
訂閱:
文章 (Atom)